mirror of
https://codeberg.org/andyscott/ziglings.git
synced 2024-11-08 19:20:47 -05:00
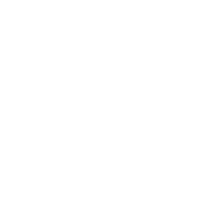
It was confusing to see tail... = undefined in the struct definition and then if (tail == null) later in the exercise - it appears that the mismatch would be the issue - but that's distracting from the real issue: making the value optional! Changing the initial value to null is still correct, but won't distract. The only worry now is that the user will remember the undefined definition from the previous exercise and wonder if that has to be that way...but you can't win them all!
46 lines
1.4 KiB
Zig
46 lines
1.4 KiB
Zig
//
|
|
// Now that we have optional types, we can apply them to structs.
|
|
// The last time we checked in with our elephants, we had to link
|
|
// all three of them together in a "circle" so that the last tail
|
|
// linked to the first elephant. This is because we had NO CONCEPT
|
|
// of a tail that didn't point to another elephant!
|
|
//
|
|
const std = @import("std"); // single quotes
|
|
|
|
const Elephant = struct {
|
|
letter: u8,
|
|
tail: *Elephant = null, // <---- make this optional!
|
|
visited: bool = false,
|
|
};
|
|
|
|
pub fn main() void {
|
|
var elephantA = Elephant{ .letter = 'A' };
|
|
var elephantB = Elephant{ .letter = 'B' };
|
|
var elephantC = Elephant{ .letter = 'C' };
|
|
|
|
// Link the elephants so that each tail "points" to the next.
|
|
elephantA.tail = &elephantB;
|
|
elephantB.tail = &elephantC;
|
|
|
|
visitElephants(&elephantA);
|
|
|
|
std.debug.print("\n", .{});
|
|
}
|
|
|
|
// This function visits all elephants once, starting with the
|
|
// first elephant and following the tails to the next elephant.
|
|
fn visitElephants(first_elephant: *Elephant) void {
|
|
var e = first_elephant;
|
|
|
|
while (!e.visited) {
|
|
std.debug.print("Elephant {u}. ", .{e.letter});
|
|
e.visited = true;
|
|
|
|
// We should stop once we encounter a tail that
|
|
// does NOT point to another element. What can
|
|
// we put here to make that happen?
|
|
if (e.tail == null) ???;
|
|
|
|
e = e.tail.?;
|
|
}
|
|
}
|